类似抖音的短视频管理系统+Java后端+vue前端(18)
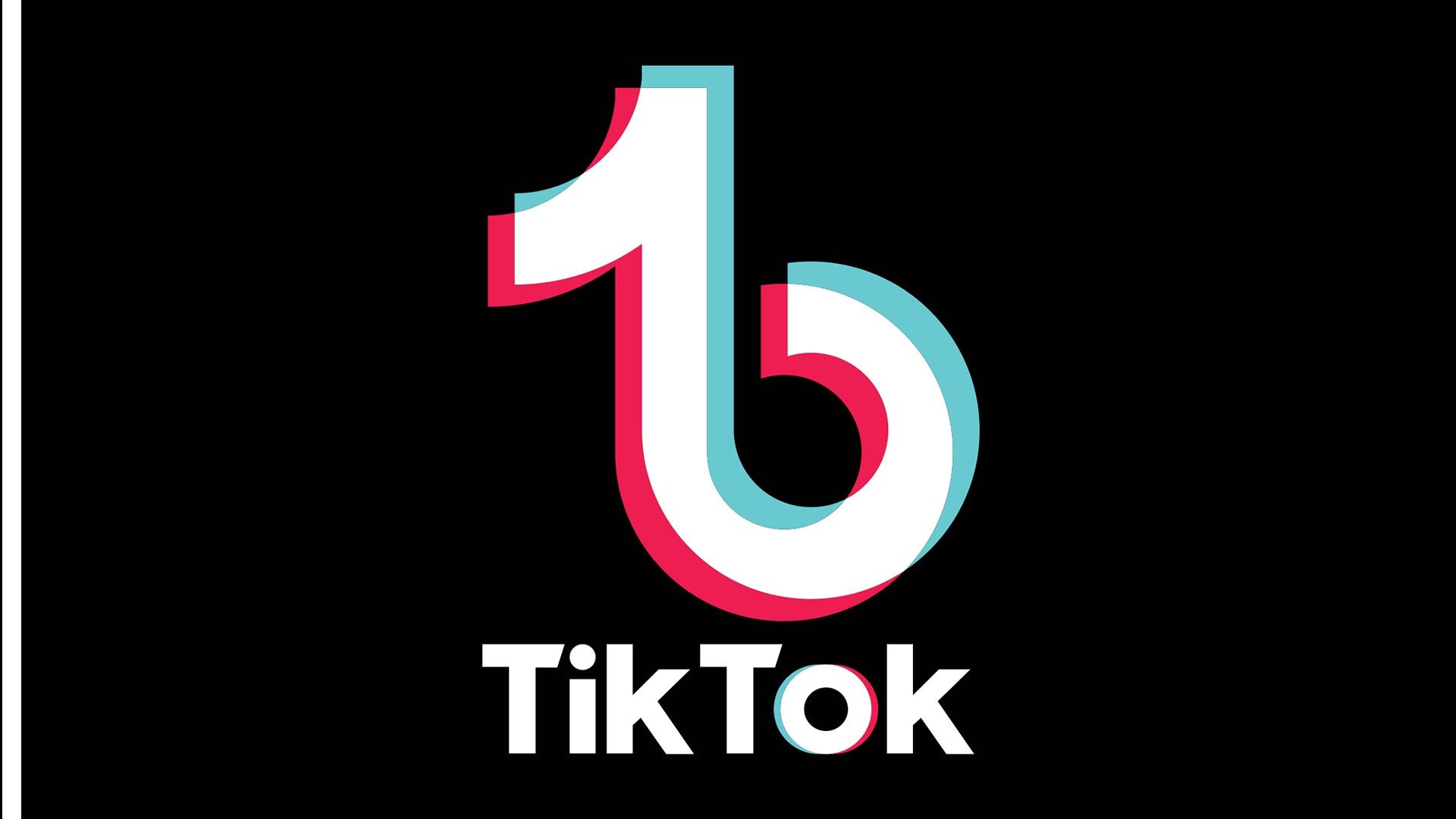
实现点击商品进入商品详情页
在路由中增加商品详情页
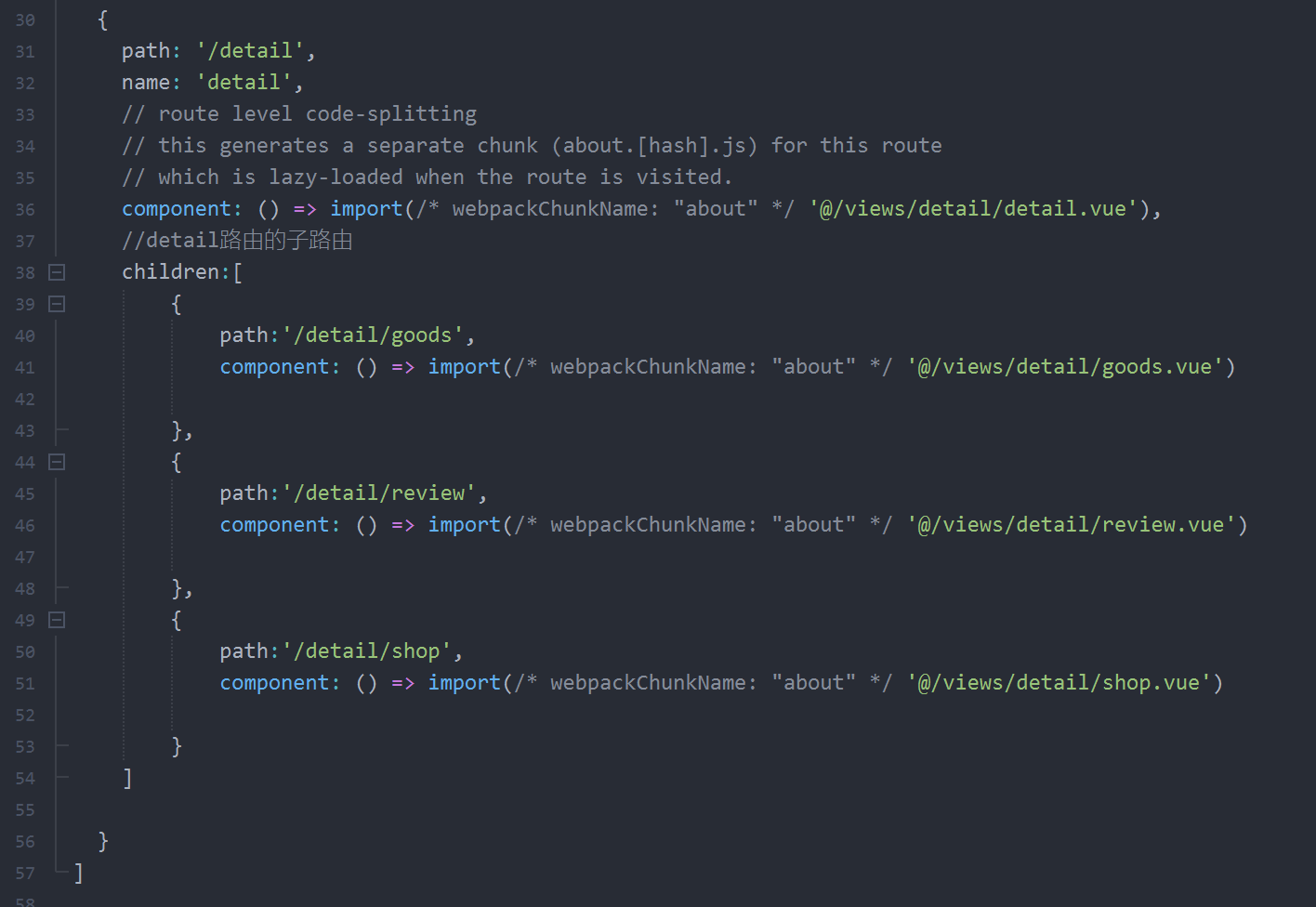
detail.vue
<template>
<div class="detail">
<img class="bg" src="../../assets/detail/chihao.jpg" alt="">
<button @click="goback()" class="back">返回</button>
<!-- 三个子组件的切换 -->
<div class="tab">
<div class="tab-item">
<router-link to="/detail/goods">商品</router-link>
</div>
<div class="tab-item">
<router-link to="/detail/review">评价</router-link>
</div>
<div class="tab-item">
<router-link to="/detail/shop">商家</router-link>
</div>
</div>
<router-view></router-view>
</div>
</template>
<script>
//调用路由
import router from '@/router/index.js'
export default{
name:"detail",
data:function(){
return {}
},
methods:{
goback:function(){
//返回首页
router.push("/")
}
},
components:{
},
mounted() {
//刚进入详情页导航栏隐藏
this.$store.commit("changeNavShow",false)
//接受列表页面传过来的索引数组
var indexs = this.$route.query
console.log(indexs)
},
//销毁页面的时候,执行函数,让底部导航栏出现
destroyed() {
this.$store.commit("changeNavShow",true)
}
}
</script>
<style>
.detail{
width: 100%;
}
.detail .bg{
width: 100%;
height: 150px;
}
.detail .back{
width: 60px;
height: 30px;
position: absolute;
top: 120px;
left: 0px;
border-radius: 20%;
border: 1px solid saddlebrown;
}
/* tab 切换的样式*/
.detail .tab{
width: 100%;
height: 30px;
display: flex;
}
.tab .tab-item{
flex: 1;
text-align: center;
background-color: whitesmoke;
line-height: 30px;
}
.tab .tab-item a{
text-decoration: none;
color: black;
}
</style>
商品列表good.vue
<template>
<div id="wrap">
<!-- 左侧 -->
<div class="goodMenu">
<ul>
<li>
<img src="../../assets/detail/baokuan.png" alt="">爆款专区
</li>
<li>
<img src="../../assets/detail/remai.png" alt="">热卖专区
</li>
<li>
<img src="../../assets/detail/wangzhe.png" alt="">王者炒饭
</li>
<li>
<img src="../../assets/detail/wangzhe.png" alt="">王者炒面
</li>
<li>
<img src="../../assets/detail/wangzhe.png" alt="">王者小吃
</li>
<li>
<img src="../../assets/detail/baokuan.png" alt="">夏日冷饮
</li>
</ul>
</div>
</div>
</template>
<script>
//导入外部的静态数据
import {getgoodList,getshopList} from "@/assets/js/data.js"
export default{
name:"goods",
data:function(){
return{
//左侧商品分类
goodList:getgoodList(),
//右侧具体商品条目
shopList:getshopList(),
//滚动坐标
scrollY:0
}
}
}
</script>
<style>
#warp{
width: 100%;
display: flex;
height: 560px;
margin-top: 20px;
overflow: hidden;
position: fixed;
}
#warp .goodMenu{
height: 80%;
width: 20%;
flex: 1;
background-color: antiquewhite;
}
.goodMenu ul{
width: 100%;
height: 80%;
list-style: none;
}
.goodMenu ul li{
height: 40px;
font-size: 15px;
width: 100%;
line-height: 40px;
}
.goodMenu ul li img{
width: 30px;
height: 30px;
position: relative;
top: 6px;
border-radius: 50%;
}
</style>