类似抖音的短视频管理系统+Java后端+vue前端(16)
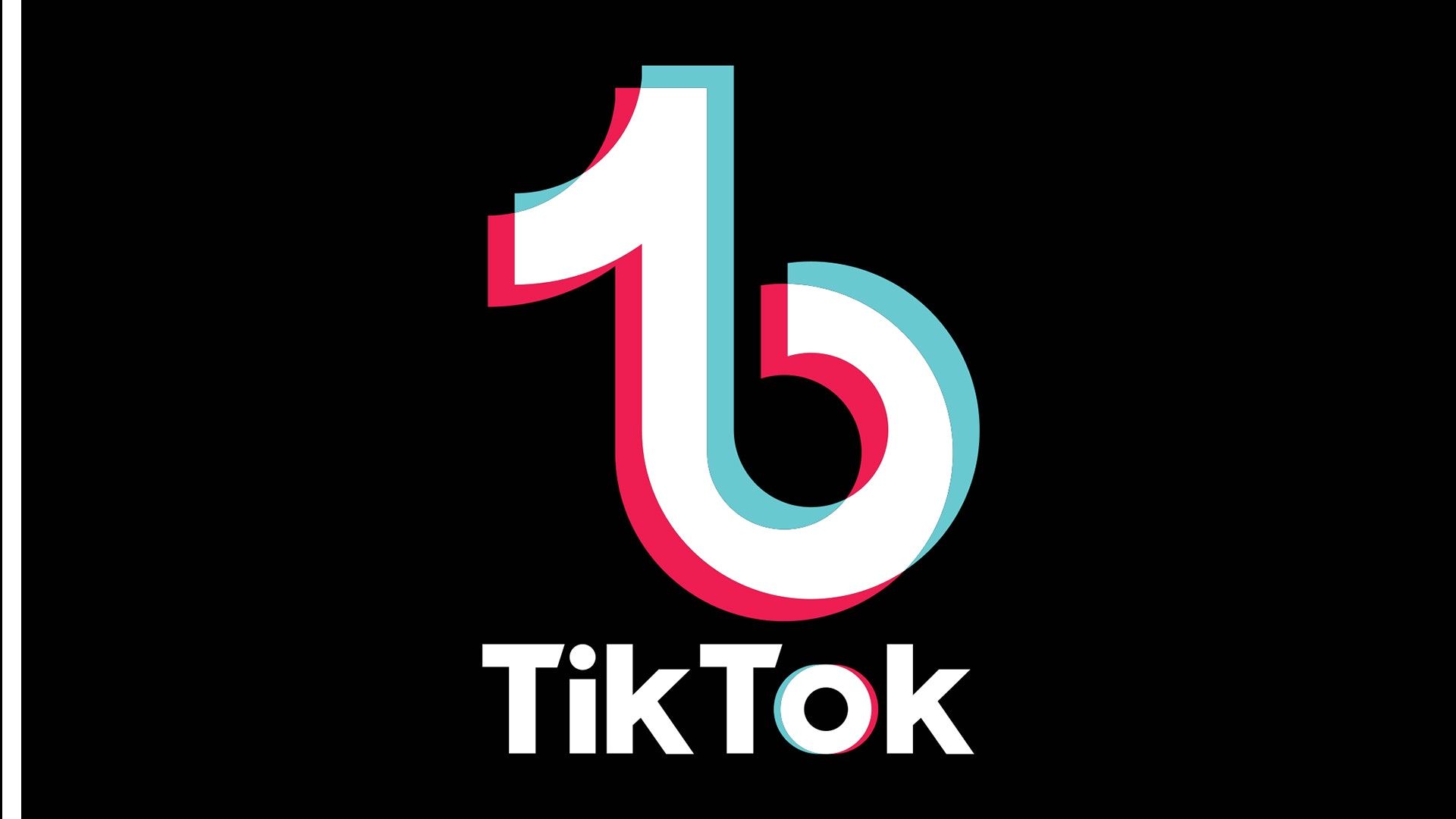
外卖项目首页搭建
前期准备
安装swiper
官网:https://3.swiper.com.cn/usage/index.html
npm install -i [email protected] -s
编写路由
在src目录下新建router目录,并新建index.js
在views下新建三个vue,分别是Home、Order、My
import Vue from 'vue'
import VueRouter from 'vue-router'
import HomeView from '../views/HomeView.vue'
import Home from '../views/Home.vue'
Vue.use(VueRouter)
const routes = [
{
path: '/',
name: 'home',
component: Home
},
{
path: '/order',
name: 'order',
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '../views/Order.vue')
},
{
path: '/my',
name: 'my',
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '../views/My.vue')
}
]
const router = new VueRouter({
mode: 'history',
base: process.env.BASE_URL,
routes
})
export default router
底部主栏
新建NavButtom.vue
<template>
<div class="nav-bottom">
<router-link to="/">
<i>首页</i>
</router-link>
<router-link to="/order">
<i>订单</i>
</router-link>
<router-link to="/my">
<i>我的</i>
</router-link>
</div>
</template>
<script>
</script>
<style>
.nav-bottom{
width:100%;
height:40px;
background-color: antiquewhite;
position: fixed;
bottom: 0;
display: flex;
}
.nav-bottom a{
display: block;
width:33%;
flex:1;
text-decoration: none;
line-height: 40px;
text-align: center;
/* width:200px; */
}
</style>
在App.vue里引入NavButtom
<template>
<div id="app">
<NavBottom></NavBottom>
<router-view/>
</div>
</template>
<script>
import NavBottom from "@/components/NavButtom.vue"
export default{
"name":"app",
data:function(){
return{
}
},
components:{
NavBottom
}
}
</script>
<style>
*{
margin:0;
padding:0;
}
</style>
效果
轮播图
新建Banner.vue
<template>
<div class="banner">
<!-- 绑定src属性 -->
<!-- <img :src="imgs[myindex]" /> -->
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide">
<img :src="imgs[0]" alt="">
</div>
<div class="swiper-slide">
<img :src="imgs[1]" alt="">
</div>
<div class="swiper-slide">
<img :src="imgs[2]" alt="">
</div>
</div>
<!-- 如果需要分页器 -->
<div class="swiper-pagination"></div>
<!-- 如果需要导航按钮 -->
<div class="swiper-button-prev"></div>
<div class="swiper-button-next"></div>
<!-- 如果需要滚动条 -->
<div class="swiper-scrollbar"></div>
</div>
</div>
</template>
<script>
import Swiper from 'swiper'
import 'swiper/dist/css/swiper.css'
export default{
name:"banner",
data:function(){
return {
imgs:[
require("@/assets/banner/banner1.jpg"),
require("@/assets/banner/banner2.jpg"),
require("@/assets/banner/banner3.jpg")
],
myindex:0
}
},
mounted() {
var that = this
var mySwiper = new Swiper ('.swiper-container', {
// 图片的滚动方向
// direction: 'vertical',
speed:1000,
autoplay:{
delay:3000
},
autoplay:true,
loop: true,
// 如果需要分页器
pagination:{
el:'.swiper-pagination'
},
// 如果需要前进后退按钮
navigation: {
nextEl:'.swiper-button-next',
prevEl:'.swiper-button-prev'
},
// 如果需要滚动条
scrollbar: {
el:'.swiper-scrollbar'
},
})
}
}
</script>
<style>
.banner{
width:100%;
/* position: fixed; */
}
img{
width:100%;
height: 150px;
}
</style>
效果
推荐
新建catogery.vue
<template>
<div class="catogery">
<ul>
<li v-for="(item,index) in subjects">
<img :src="item.pic" alt="" @click="changeType(index)">
<div>
<label for="">{{item.subject}}</label>
</div>
</li>
</ul>
</div>
</template>
<script>
export default{
name:"catogery",
data:function(){
return{
subjects:[
{subject:"热门",pic:require('@/assets/catogery/hot.png')}
, {subject:"披萨",pic:require('@/assets/catogery/liulian.jpg')}
, {subject:"皮蛋瘦肉粥",pic:require('@/assets/catogery/zhou.jpg')}
, {subject:"饺子馄饨",pic:require('@/assets/catogery/jiaozi.jpg')}
, {subject:"披萨",pic:require('@/assets/catogery/liulian.jpg')}
, {subject:"皮蛋瘦肉粥",pic:require('@/assets/catogery/zhou.jpg')}
]
}
},
methods:{
changeType:function(data){
console.log("....."+data)
}
}
}
</script>
<style>
.catogery{
/* margin-top: 150px; */
width: 100%;
height: 140px;
background: whitesmoke;
position: absolute;
}
.catogery ul{
display: flex;
flex-wrap: wrap;
font-size: 12px;
justify-content: space-around;
}
.catogery ul li{
width: 30%;
text-align: center;
list-style: none;
}
.catogery ul li img{
width: 45px;
height: 45px;
border-radius: 20%;
}
</style>
在home中引入Banner和catogery
<template>
<div class="home">
<Banner></Banner>
<Catogery></Catogery>
<router-view/>
</div>
</template>
<script>
import Banner from "@/components/Banner.vue"
import Catogery from "@/components/catogery.vue"
export default{
"name":"home",
data:function(){
return{
}
},
components:{
Banner,
Catogery
}
}
</script>
<style>
.home{
height: 900px;
}
</style>
效果