类似抖音的短视频管理系统+Java后端+vue前端(14)
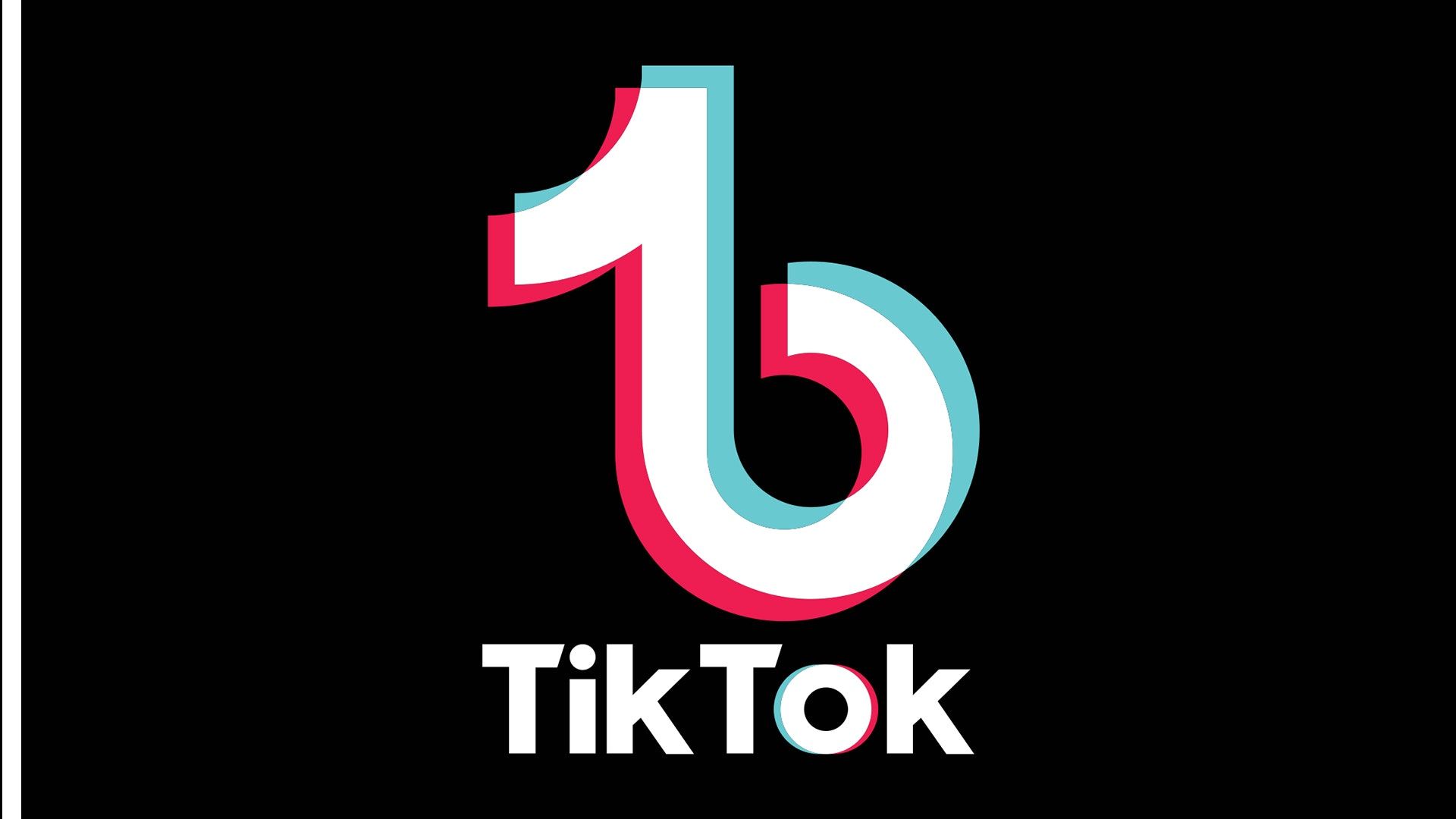
vue基础语法
vue项目框架
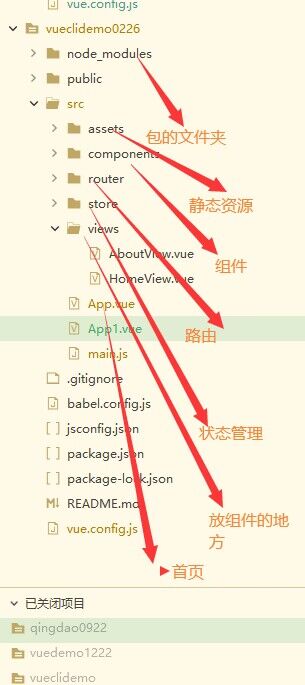
新建vue.config.js
let path = require('path')
const {defineConfig} = require('@vue/cli-service')
module.exports = defineConfig({
devServer: {
open: true,
host: 'localhost',
port: '9999',
historyApiFallback:true,
allowedHosts:"all",
client:{
webSocketURL:'ws://0.0.0.0:9999/ws'
},
headers:{
'Access-Control-Allow-Origin':'*'
},
proxy: {
'/': {
target: 'http://localhost:3000', // 要请求springboot 后端的地址
changeOrigin: true,
pathRewrite: {
'^/': '/'
},
ws:false
}
} //解决跨域问题
},
configureWebpack:(config)=>{
config.resolve = {
extensions:['.js','.json','.vue'],
alias:{
'@':path.resolve(__dirname,'./src')
}
}
}
});
新建App1.vue并在main.js中添加
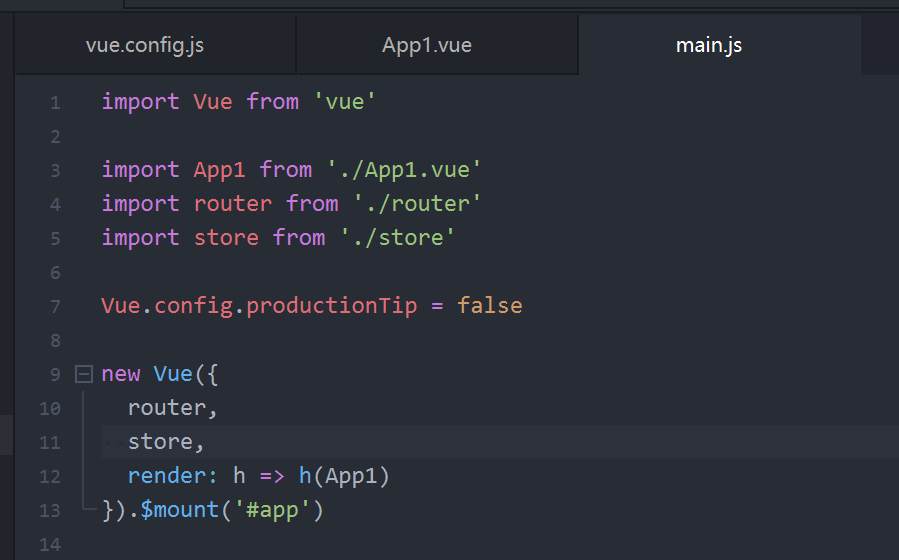
<template>
<div id="app">
<!-- {{插值表达式}} -->
<!-- 以vue结尾的叫组件 -->
<h1>{{msg}}</h1>
<ul>
<!-- v-for 循环遍历数组 -->
<li v-for="item in array">
{{item}}
</li>
</ul>
<!-- v-if 控制标签的显示与消失 -->
<img v-if="flag" :src="picUrl" id="img1"/>
<!-- v- 控制标签的显示与隐藏 -->
<img v-show="flag" :src="picUrl1"/>
<!-- vue中的点击事件 -->
<button v-on:click="flag =! flag">显示与隐藏</button>
<button @click="flag =! flag">点击事件简写方式</button>
<!-- v-html可以将标签元素嵌入到元素当中 -->
<!-- v-bind:class样式属性绑定,样式是否存在由isActive决定-->
<div v-html="myhtml1" v-bind:class="{myborder:isActive}">
234234
</div>
<button @click="myAlert()">点击弹出窗口</button>
<!-- 实现双向数据绑定 -->
<!-- v-model是语法优化 -->
<!-- @keydown.enter 按下回车 -->
<br />
{{msg1}}
<br />
<input v-model="msg1" @keydown.enter="myAlert()"/>
<br/>
{{reverseMsg}}
<br />
<button @click="getJoke()">笑话</button>
<table border="1">
<tr v-for="joke in msg2">
<td>
{{joke}}
</td>
</tr>
</table>
<br />
<button @click="getVideo()">笑话</button>
<table border="1">
<tr v-for="video in msg3">
<td>
{{video}}
</td>
</tr>
</table>
</div>
</template>
<script>
//vue基础
export default{
name:'app1',
data:function(){
return{
msg:"qweqweqwe",
array:['html','云计算','网络安全','大数据','java'],
flag:true,
myhtml:"<h2>白龙马蹄朝西</h2>",
myhtml1:"<img :src='picUrl'>",
msg1:"白龙马蹄朝西",
isActive:true,
//require 引入图片 @ 代表根路径
picUrl:require('@/assets/tiktok.png'),
picUrl1:require('@/assets/logo.png'),
msg2:'',
msg3:''
}
},
methods:{
myAlert:function(){
// alert("点点点点点点")
var img = document.getElementById("img1");
img.style.width = img.offsetWidth +5 +"px";
},
getJoke:function(){
//通过'axios将msg2的值换掉
//this在then里面的function没有意义,所以需要将它赋值给that
var that = this
this.$http.get("https://autumnfish.cn/api/joke/list?num=5").then(function(res){
console.log(res)
that.msg2 = res
})
},
getVideo:function(){
//通过'axios将msg2的值换掉
//this在then里面的function没有意义,所以需要将它赋值给that
var that = this
axios({
type:"get",
url:"/videos/showAll",
method:"get",
hraders:{
'content-type':'application/x-www-form-urlencoded'
}
}).then(function(res){
console.log(res)
that.msg3 = res
})
}
},
computed:{
reverseMsg:function(msg1){
// split("")变成数组,reverse()反翻转,join("")变成字符串
return this.msg1.split("").reverse().join("")
}
}
}
</script>
<style>
.myborder{
width: 200px;
height: 200px;
border: 1px black solid;
}
img{
width: 100px;
}
</style>
main.js
import Vue from 'vue'
import App1 from './App1.vue'
import router from './router'
import store from './store'
//axios 相对与ajax
import axios from 'axios'
Vue.config.productionTip = false
//相当与vuecli中的全局变量
Vue.prototype.$http = axios
new Vue({
router,
store,
render: h => h(App1)
}).$mount('#app')
需要在项目node_moudules下安装axios
axios相当于ajax
npm install -i axios -S